Applying SOLID Principles in Software Development
Applying SOLID Principles in Software Development
Introduction
Software development is a discipline that demands quality, scalability, and long-term maintenance. To achieve this, the SOLID principles have emerged as a set of essential best practices. These principles guide developers to write cleaner, more flexible, and easier-to-modify code, which is crucial in complex software projects.
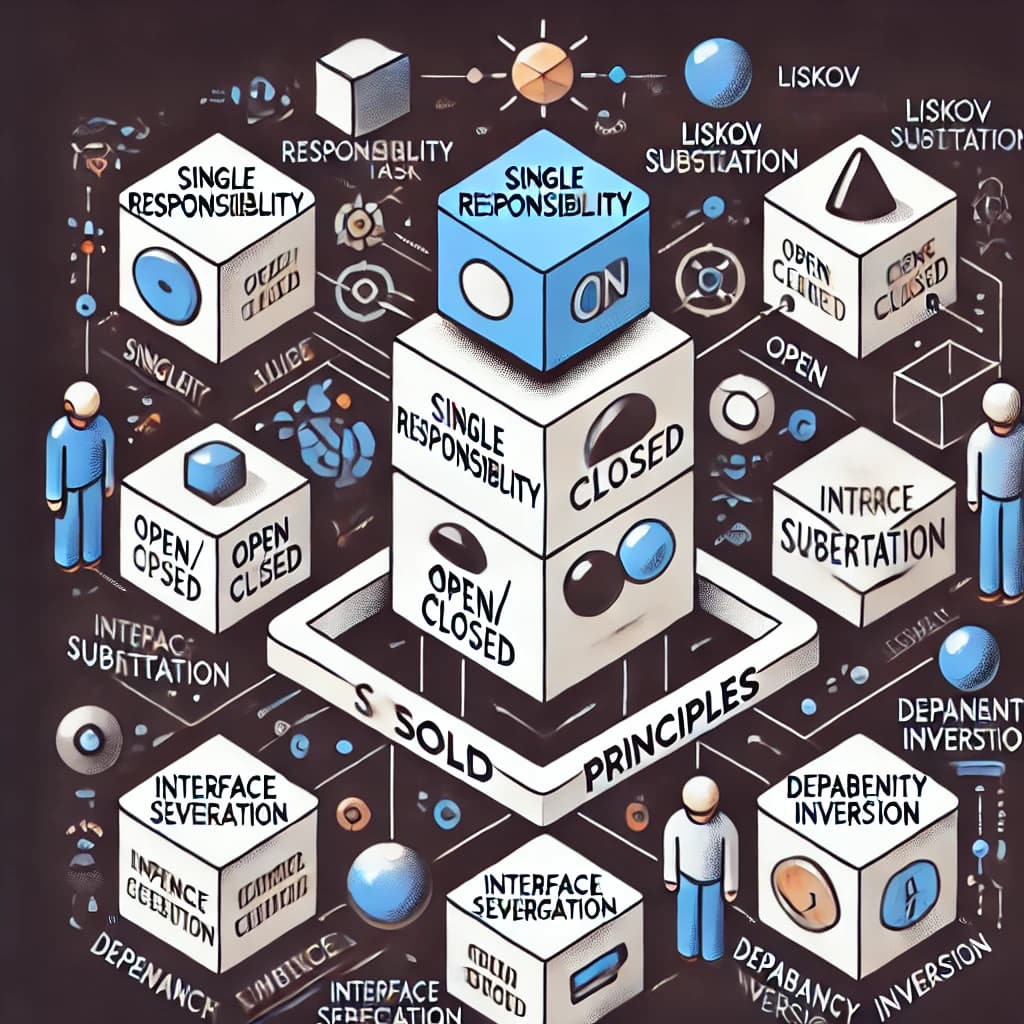
The SOLID principles, an acronym of five foundational concepts, were introduced by Robert C. Martin. These principles aim to solve common problems in object-oriented programming, such as tight code dependencies, difficulty in adding new features, and lack of clarity in class and method design.
The Five SOLID Principles
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change, meaning it should only be responsible for one task. This principle is crucial to avoid classes with multiple responsibilities, which become difficult to understand, maintain, and test.
Scientific Application:
By following SRP, code becomes more modular, with each component specializing in a single responsibility. This reduces coupling between components and allows modifying or updating one part of the system without affecting others. In scientific terms, this is a manifestation of high cohesion, improving system maintainability and scalability.
2. Open/Closed Principle (OCP)
The Open/Closed Principle states that a class should be open for extension but closed for modification. This means that we should be able to add new functionality without modifying existing code, thus avoiding introducing errors into parts of the system that already work correctly.
Scientific Application:
OCP encourages the use of inheritance and composition as mechanisms to extend class behavior without modifying it directly. From a theoretical perspective, this principle facilitates the controlled evolution of software systems, preventing the unintended propagation of changes across the entire system.
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a derived class should be able to replace objects of the base class without altering the functionality of the system. In other words, subclasses should be able to replace their base classes without introducing unexpected behaviors.
Scientific Application:
This principle ensures that class hierarchies are consistent, promoting correct inheritance and preventing the introduction of subtypes that do not meet the expected behavior. LSP is based on the theory of subtyping, ensuring that subclasses do not violate the expectations set by the base class.
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle states that it's better to have multiple specific interfaces than a single general-purpose interface. This way, clients are not forced to implement methods they don’t need.
Scientific Application:
ISP reflects the principle of specialization in software design, which reduces coupling and improves cohesion. From a mathematical perspective, it ensures that system modules have clear interfaces that describe their behavior in terms of a limited set of operations.
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules, but both should depend on abstractions. Furthermore, abstractions should not depend on details, but details should depend on abstractions.
Scientific Application:
DIP promotes decoupling dependencies between modules, which is crucial for building flexible and easily adaptable systems. This principle follows the theory of abstraction and generalization, allowing systems to be designed with less dependency on concrete implementations and, therefore, easier to test and maintain.
Implementing SOLID in Real Development
Applying the SOLID principles can make a significant difference in the quality of the software. By following these principles, developers create modular, scalable, and easily extendable systems. Systems that follow SOLID principles are easier to maintain and less prone to issues as they grow.
Conclusion
The SOLID principles are fundamental for writing quality code in large-scale software projects. Implementing these principles not only improves the maintainability and scalability of code but also encourages clean, modular design that makes extending and modifying software without introducing unintended side effects much easier.
Understanding and correctly applying SOLID provides a scientific framework that allows building more robust, easier-to-understand systems, and adaptable to the changing needs of users and businesses.